이번 시간에는 설정화면에서 배경음악 ON/OFF 기능을 만들어 보겠습니다.
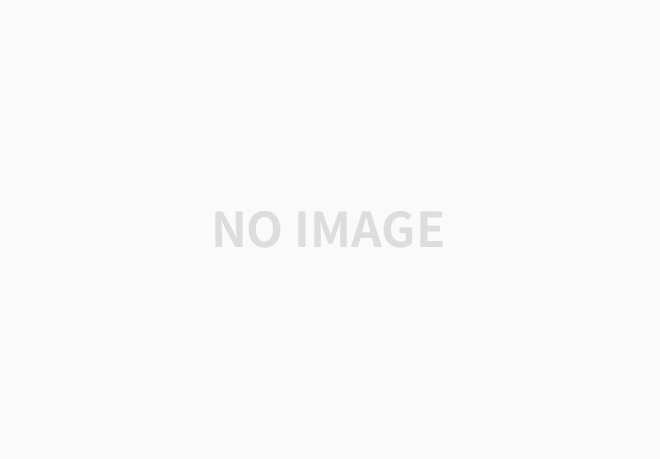
아래 mp4파일을 다운받으시면 사운드가 적용된 결과를 보실 수 있습니다.
자바 파일 3개와 xml파일 2개 그리고 음악파일 1개가 필요합니다.
<자바 파일>
MainActivity
MusicService
Setting
<xml 파일>
activity_main
activity_setting
<mp3 파일>
mp3파일은 res 하위에 raw폴더를 만들고 그 안에 넣어놓습니다.
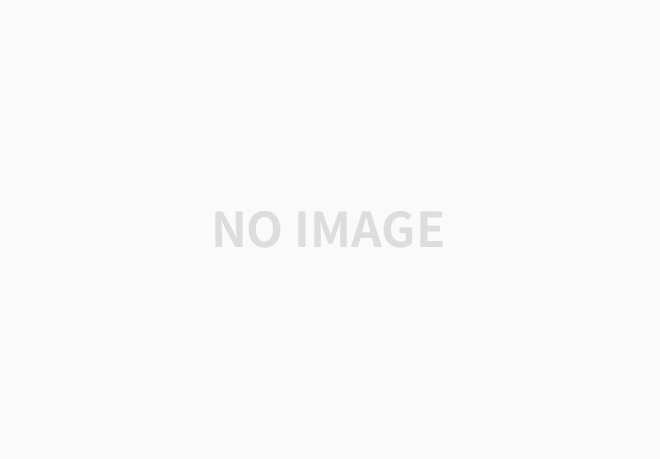
▶스틱코드 홈페이지
https://stickode.com/mainlogin.html
배경음악 코드와 스위치버튼 코드, 그리고 서비스 상태 확인 코드를 스틱코드에서 가져옵니다.
1. 배경 음악 코드
https://stickode.com/detail.html?no=1884
여기서 배경음악 코드를 즐겨찾기 하고 안드로이드 스튜디오에서 MusicService를 입력하면
손쉽게 코드를 불러 올 수 있습니다. (MusicService는 해당 포스팅의 태그 입니다.)
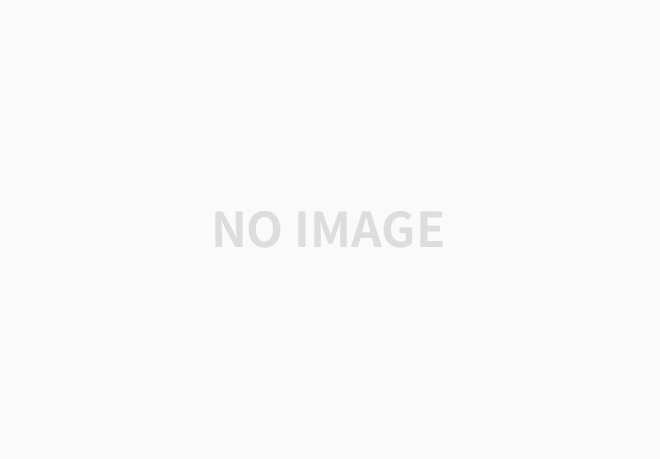
같은 방법으로 나머지 코드들을 입력합니다.
2. 스위치버튼 코드
https://stickode.com/detail.html?no=649
3. 서비스 상태 확인 코드
https://stickode.com/detail.html?no=1278
위 코드들을 조합하여 완성하면 아래와 같습니다.
1. MainActivity.java
public class MainActivity extends AppCompatActivity {
public static boolean SoundCheck = true; //앱이 시작되면 음악 스위치를 켠다.
String class_name = MusicService.class.getName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button Btn_Setting = (Button)findViewById(R.id.Btn_Setting);
Btn_Setting.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MainActivity.this,Setting.class);
startActivity(intent);
}
});
boolean isServiceRunningCheck = isServiceRunningCheck(class_name);
if(SoundCheck && !isServiceRunningCheck) { //스위치가 켜져있는데 서비스가 꺼져 있으면 음악을 킨다.
Intent intent1 = new Intent(MainActivity.this, MusicService.class);
startService(intent1);
}
}
public boolean isServiceRunningCheck(String class_name) {
ActivityManager manager = (ActivityManager) this.getSystemService(Activity.ACTIVITY_SERVICE);
for (ActivityManager.RunningServiceInfo service : manager.getRunningServices(Integer.MAX_VALUE)) {
if (class_name.equals(service.service.getClassName())) {
return true;
}
}
return false;
}
}
* 홈화면으로 돌아왔을 때 서비스 상태를 확인하고
만약 스위치가 켜져있는데 서비스가 꺼져 있으면 음악을 키도록 코드를 작성합니다.
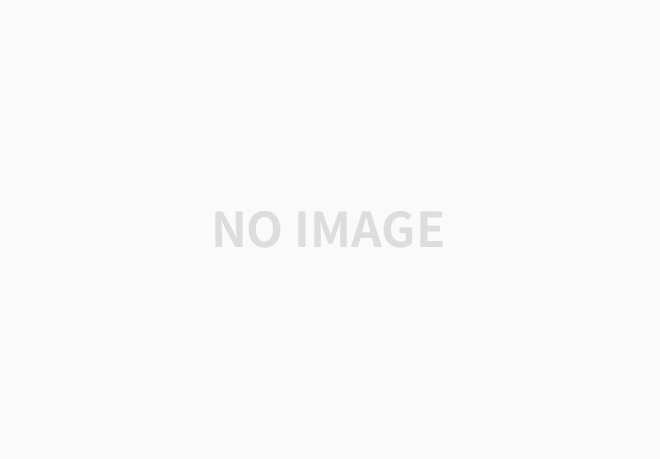
2. MusicService.java
public class MusicService extends Service
{
private MediaPlayer player;
public MusicService()
{
}
@Override
public IBinder onBind(Intent intent)
{
return null;
}
@Override
public void onCreate()
{
Log.e("음악 재생 서비스", "onCreate() 호출");
super.onCreate();
}
@Override
public void onDestroy()
{
Log.e("음악 재생 서비스", "onDestroy() 호출");
player.stop();
super.onDestroy();
}
@Override
public int onStartCommand(Intent intent, int flags, int startId)
{
Log.e("서비스 테스트", "onStartCommand() 호출");
player = MediaPlayer.create(this, R.raw.turned_to_dust);
player.setLooping(true);
player.start();
return super.onStartCommand(intent, flags, startId);
}
}
R.raw.____ 이 부분에 미리 넣어둔 mp3파일 제목을 입력합니다. 대문자나 특수문자가 들어가지 않도록 주의해주세요.
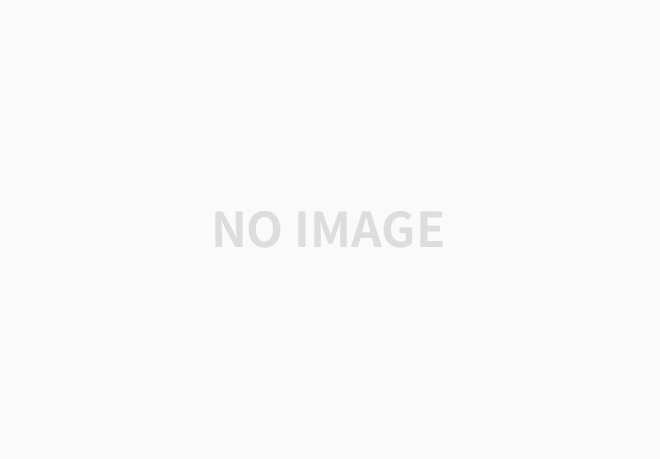
3. Setting.java
public class Setting extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_setting);
Button Btn_Home = (Button)findViewById(R.id.Btn_Home);
Switch switchButton = findViewById(R.id.switch1);
if(MainActivity.SoundCheck){
switchButton.setChecked(true);}
else{
switchButton.setChecked(false);
}
Btn_Home.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(Setting.this,MainActivity.class);
startActivity(intent);
}
});
switchButton.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if (isChecked) {
MainActivity.SoundCheck = true;
} else {
MainActivity.SoundCheck = false;
Intent intent2 = new Intent(Setting.this, MusicService.class);
stopService(intent2);
}
}
});
}
}
4. activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/Btn_Setting"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginEnd="36dp"
android:layout_marginBottom="68dp"
android:text="설정화면으로 가기"
android:textSize="40dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
5. activity_setting.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".Setting">
<Button
android:id="@+id/Btn_Home"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="116dp"
android:text="홈으로 가기"
android:textSize="50dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintStart_toStartOf="parent" />
<Switch
android:id="@+id/switch1"
android:layout_width="158dp"
android:layout_height="66dp"
android:layout_marginStart="124dp"
android:layout_marginTop="132dp"
android:text="Sound"
android:textSize="25dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
'안드로이드 자바' 카테고리의 다른 글
[JAVA][Android] 로또 QR 코드 웹뷰 띄워주기 (0) | 2021.06.08 |
---|---|
[JAVA][Android] 안드로이드 STT (4) | 2021.06.06 |
[JAVA][Android] Animation을 활용한 하트 ToggleButton (2) | 2021.05.29 |
[Java][Android] 안드로이드 TTS (0) | 2021.05.26 |
[JAVA][Android] 핸들러를 활용한 타이머 구현하기 (0) | 2021.05.22 |