728x90
이번 시간에는 intent를 사용하여 이미지를 다른 화면으로 보내보겠습니다.
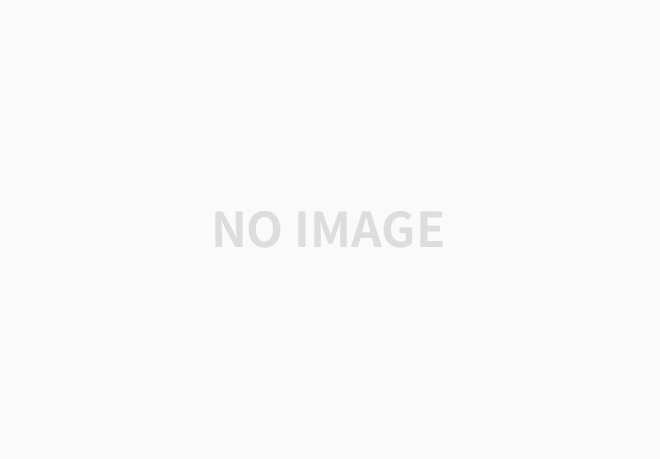
우선 사진 한 장과 화면 두 개가 필요하겠죠?
메인 액티비티와 세컨드 액티비티를 준비해주세요.
사진 이미지는 drawble 폴더에 jpg 파일을 넣어줍니다.
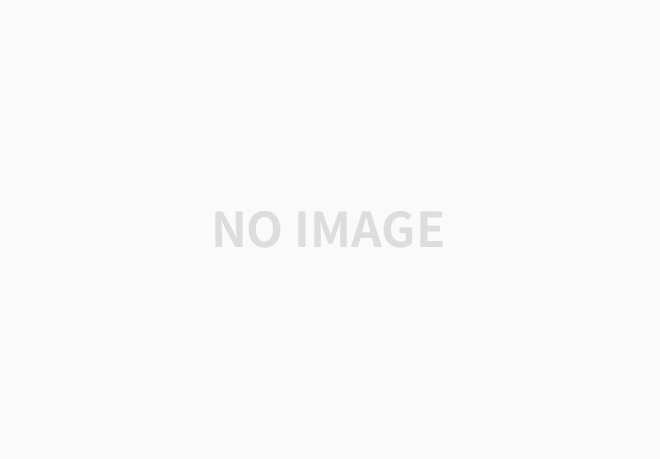
그리고 스틱코드에서 파일 4개를 손쉽게 가져옵니다.
https://stickode.com/mainlogin.html
스틱코드의 여러 포스팅 중에서 아래 링크의 포스팅을 즐겨찾기 합니다.
https://stickode.com/detail.html?no=2166
1. MainActivity.java
즐겨찾기 해놓은 코드를 태그만 입력해서 모두 불러 올 수 있습니다.
태그를 입력하여 해당 코드를 더블 클릭하면 즐겨찾기 해놓은 코드가 생성됩니다.
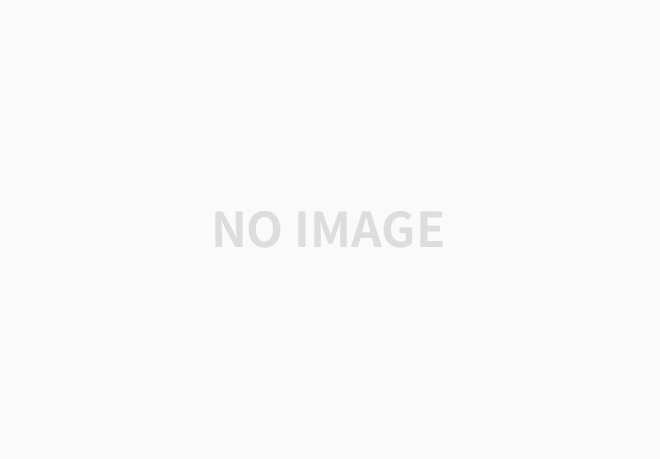
public class MainActivity extends AppCompatActivity {
ImageView imageview;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageview = (ImageView)findViewById(R.id.imageView);
Button button = (Button)findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
ByteArrayOutputStream stream = new ByteArrayOutputStream();
Bitmap bitmap = ((BitmapDrawable)imageview.getDrawable()).getBitmap();
float scale = (float) (1024/(float)bitmap.getWidth());
int image_w = (int) (bitmap.getWidth() * scale);
int image_h = (int) (bitmap.getHeight() * scale);
Bitmap resize = Bitmap.createScaledBitmap(bitmap, image_w, image_h, true);
resize.compress(Bitmap.CompressFormat.JPEG, 100, stream);
byte[] byteArray = stream.toByteArray();
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
intent.putExtra("image", byteArray);
startActivity(intent);
}
});
}
}
2. SecondActivity.java
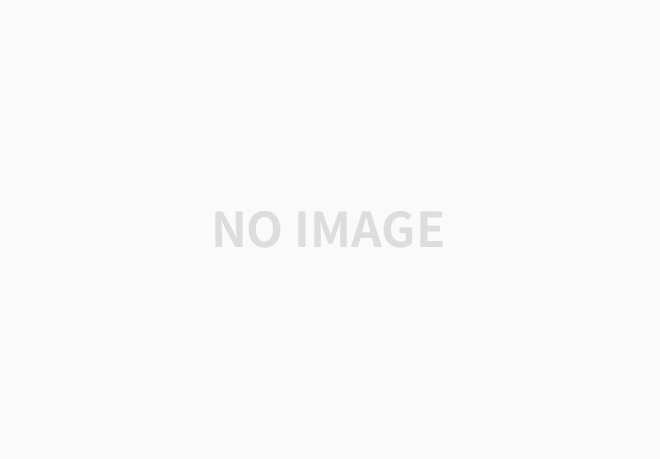
public class SecondActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
ImageView imageview = (ImageView)findViewById(R.id.imageView);
byte[] byteArray = getIntent().getByteArrayExtra("image");
Bitmap bitmap = BitmapFactory.decodeByteArray(byteArray, 0, byteArray.length);
imageview.setImageBitmap(bitmap);
}
}
3. activity_main.xml
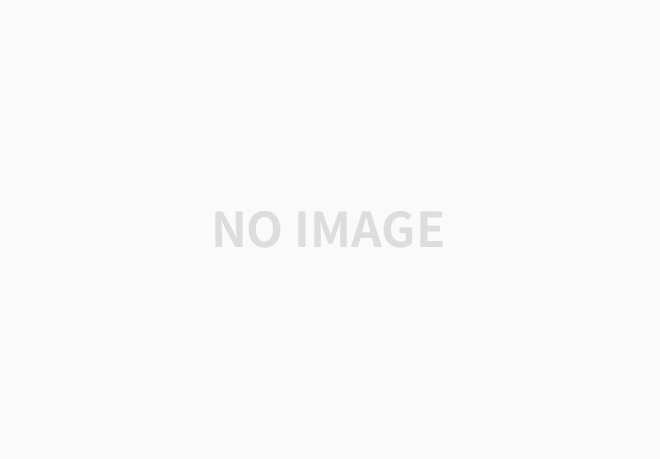
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="200dp"
android:src="@drawable/bg"/>
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="사진 설명"/>
</LinearLayout>
4. activity_second.xml
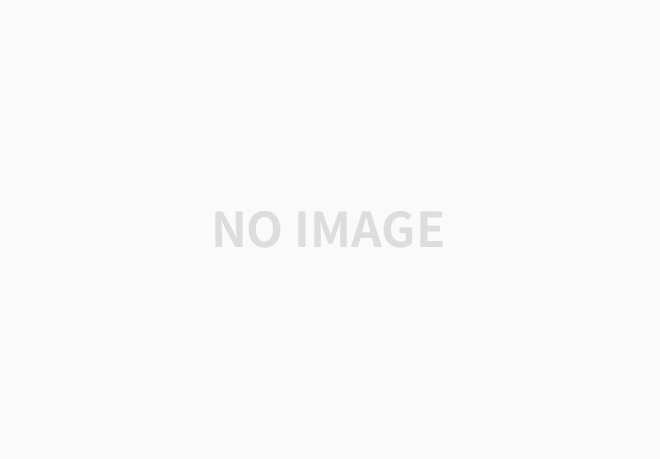
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical"
tools:context=".SecondActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="200dp" />
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum." />
</LinearLayout>
'안드로이드 자바' 카테고리의 다른 글
[Java][Android] MLkit를 이용한 얼굴 탐지 (0) | 2021.06.19 |
---|---|
[JAVA][Android] 달력 빠르게 만들기 (0) | 2021.06.18 |
[JAVA][Android] 안드로이드 OCR 기능 만들기 (4) | 2021.06.13 |
[JAVA][Android] 라이브 방송 화면 만들기 (0) | 2021.06.11 |
[JAVA][android] WiFi정보 스캔 빠르게 구현하기 (0) | 2021.06.09 |